What needs to be changed in this archiving script to only archive specific file types?
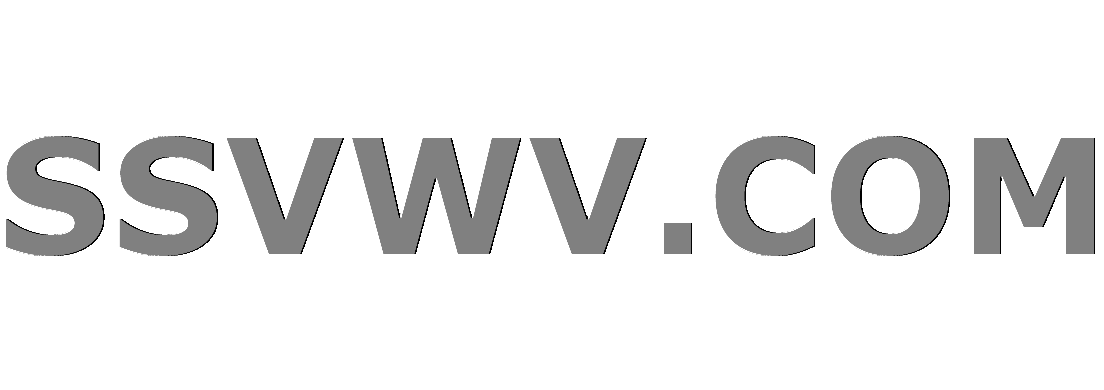
Multi tool use
up vote
0
down vote
favorite
I made this script based on past answers from superuser, but now need to only archive specific file types. I messed around trying to use wildcards but nothing I tried seemed to stick.
If I wanted to change this script to filter by specific file-types instead of all file system objects, what needs to be changed and how? For example, if I wanted to only archive DLL files, or DLL & EXE files, how would it be changed?
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
command-line compression archiving vbscript
add a comment |
up vote
0
down vote
favorite
I made this script based on past answers from superuser, but now need to only archive specific file types. I messed around trying to use wildcards but nothing I tried seemed to stick.
If I wanted to change this script to filter by specific file-types instead of all file system objects, what needs to be changed and how? For example, if I wanted to only archive DLL files, or DLL & EXE files, how would it be changed?
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
command-line compression archiving vbscript
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I made this script based on past answers from superuser, but now need to only archive specific file types. I messed around trying to use wildcards but nothing I tried seemed to stick.
If I wanted to change this script to filter by specific file-types instead of all file system objects, what needs to be changed and how? For example, if I wanted to only archive DLL files, or DLL & EXE files, how would it be changed?
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
command-line compression archiving vbscript
I made this script based on past answers from superuser, but now need to only archive specific file types. I messed around trying to use wildcards but nothing I tried seemed to stick.
If I wanted to change this script to filter by specific file-types instead of all file system objects, what needs to be changed and how? For example, if I wanted to only archive DLL files, or DLL & EXE files, how would it be changed?
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
command-line compression archiving vbscript
command-line compression archiving vbscript
edited Nov 13 at 1:03
asked Nov 13 at 0:39
kayleeFrye_onDeck
1508
1508
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
You can use a filter. The magic is here:
SHCONTF_NONFOLDERS = 64
colFolderItems.Filter SHCONTF_NONFOLDERS, "*.ext"
Here it is in context with your code:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
FileFilter = parameters(2)
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
SHCONTF_NONFOLDERS = 64
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
This can be called with "*.txt" to select only text files, or "*.exe" to collect only exe files. Also note that it doesn't play well if there is no file filter included.
However, your code is designed to recreate the ZIP each time, so you'd need to test for the existence of the file first if you want to call repeatedly for additional file types. This change does that:
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
This does have a side-effect of popping (and almost immediately hiding) an overwrite prompt if the files already exist in the zip file. With that change you could do this:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.txt"
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc"
It could be changed to iterate file types or extensions and collect all files if there is no filter, as so:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern" "file2.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
SHCONTF_NONFOLDERS = 64
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
Set shell = CreateObject("Shell.Application")
If parameters.Count > 2 Then
For lParams = 2 to (parameters.Count-1)
FileFilter = parameters(lParams)
Set source_objects = shell.NameSpace(SourceDir).Items
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
Next
Else
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
End If
This could be called like:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc" "*.txt"
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You can use a filter. The magic is here:
SHCONTF_NONFOLDERS = 64
colFolderItems.Filter SHCONTF_NONFOLDERS, "*.ext"
Here it is in context with your code:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
FileFilter = parameters(2)
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
SHCONTF_NONFOLDERS = 64
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
This can be called with "*.txt" to select only text files, or "*.exe" to collect only exe files. Also note that it doesn't play well if there is no file filter included.
However, your code is designed to recreate the ZIP each time, so you'd need to test for the existence of the file first if you want to call repeatedly for additional file types. This change does that:
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
This does have a side-effect of popping (and almost immediately hiding) an overwrite prompt if the files already exist in the zip file. With that change you could do this:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.txt"
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc"
It could be changed to iterate file types or extensions and collect all files if there is no filter, as so:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern" "file2.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
SHCONTF_NONFOLDERS = 64
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
Set shell = CreateObject("Shell.Application")
If parameters.Count > 2 Then
For lParams = 2 to (parameters.Count-1)
FileFilter = parameters(lParams)
Set source_objects = shell.NameSpace(SourceDir).Items
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
Next
Else
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
End If
This could be called like:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc" "*.txt"
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
add a comment |
up vote
1
down vote
You can use a filter. The magic is here:
SHCONTF_NONFOLDERS = 64
colFolderItems.Filter SHCONTF_NONFOLDERS, "*.ext"
Here it is in context with your code:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
FileFilter = parameters(2)
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
SHCONTF_NONFOLDERS = 64
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
This can be called with "*.txt" to select only text files, or "*.exe" to collect only exe files. Also note that it doesn't play well if there is no file filter included.
However, your code is designed to recreate the ZIP each time, so you'd need to test for the existence of the file first if you want to call repeatedly for additional file types. This change does that:
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
This does have a side-effect of popping (and almost immediately hiding) an overwrite prompt if the files already exist in the zip file. With that change you could do this:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.txt"
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc"
It could be changed to iterate file types or extensions and collect all files if there is no filter, as so:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern" "file2.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
SHCONTF_NONFOLDERS = 64
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
Set shell = CreateObject("Shell.Application")
If parameters.Count > 2 Then
For lParams = 2 to (parameters.Count-1)
FileFilter = parameters(lParams)
Set source_objects = shell.NameSpace(SourceDir).Items
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
Next
Else
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
End If
This could be called like:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc" "*.txt"
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
add a comment |
up vote
1
down vote
up vote
1
down vote
You can use a filter. The magic is here:
SHCONTF_NONFOLDERS = 64
colFolderItems.Filter SHCONTF_NONFOLDERS, "*.ext"
Here it is in context with your code:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
FileFilter = parameters(2)
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
SHCONTF_NONFOLDERS = 64
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
This can be called with "*.txt" to select only text files, or "*.exe" to collect only exe files. Also note that it doesn't play well if there is no file filter included.
However, your code is designed to recreate the ZIP each time, so you'd need to test for the existence of the file first if you want to call repeatedly for additional file types. This change does that:
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
This does have a side-effect of popping (and almost immediately hiding) an overwrite prompt if the files already exist in the zip file. With that change you could do this:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.txt"
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc"
It could be changed to iterate file types or extensions and collect all files if there is no filter, as so:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern" "file2.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
SHCONTF_NONFOLDERS = 64
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
Set shell = CreateObject("Shell.Application")
If parameters.Count > 2 Then
For lParams = 2 to (parameters.Count-1)
FileFilter = parameters(lParams)
Set source_objects = shell.NameSpace(SourceDir).Items
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
Next
Else
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
End If
This could be called like:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc" "*.txt"
You can use a filter. The magic is here:
SHCONTF_NONFOLDERS = 64
colFolderItems.Filter SHCONTF_NONFOLDERS, "*.ext"
Here it is in context with your code:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
FileFilter = parameters(2)
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
Set shell = CreateObject("Shell.Application")
Set source_objects = shell.NameSpace(SourceDir).Items
SHCONTF_NONFOLDERS = 64
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
This can be called with "*.txt" to select only text files, or "*.exe" to collect only exe files. Also note that it doesn't play well if there is no file filter included.
However, your code is designed to recreate the ZIP each time, so you'd need to test for the existence of the file first if you want to call repeatedly for additional file types. This change does that:
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
This does have a side-effect of popping (and almost immediately hiding) an overwrite prompt if the files already exist in the zip file. With that change you could do this:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.txt"
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc"
It could be changed to iterate file types or extensions and collect all files if there is no filter, as so:
'To use this at command-line, call `CScript.exe zip_it.vbs SourceDirectory PathToOutputZipFileIncludingDotZipExt "file.pattern" "file2.pattern"`
Set parameters = WScript.Arguments
Set FS = CreateObject("Scripting.FileSystemObject")
SourceDir = FS.GetAbsolutePathName(parameters(0))
ZipFile = FS.GetAbsolutePathName(parameters(1))
SHCONTF_NONFOLDERS = 64
If Not FS.FileExists (ZipFile) Then
CreateObject("Scripting.FileSystemObject").CreateTextFile(ZipFile, True).Write "PK" & Chr(5) & Chr(6) & String(18, vbNullChar)
End If
Set shell = CreateObject("Shell.Application")
If parameters.Count > 2 Then
For lParams = 2 to (parameters.Count-1)
FileFilter = parameters(lParams)
Set source_objects = shell.NameSpace(SourceDir).Items
source_objects.Filter SHCONTF_NONFOLDERS, FileFilter
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
Next
Else
Set source_objects = shell.NameSpace(SourceDir).Items
shell.NameSpace(ZipFile).CopyHere(source_objects)
wScript.Sleep 400
End If
This could be called like:
cscript //nologo zip_it.vbs thefolder thefile.zip "*.doc" "*.txt"
answered Nov 21 at 11:06
shawn
765
765
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
add a comment |
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
I like your changes, but unfortunately as-written it's falling a little short. For one, it can only handle file-filters if that specific file-type exists directly in the source directory sent as the parameter. For another, even if it does, it won't find any in sub-directories. Interestingly enough, neither of our scripts seem to handle huge directories recursively; only small ones which is distressing! I didn't realize mine was so weak :(
– kayleeFrye_onDeck
Nov 22 at 4:37
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsuperuser.com%2fquestions%2f1374872%2fwhat-needs-to-be-changed-in-this-archiving-script-to-only-archive-specific-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cOYmj7,Za qhXUrCD7XYXwkrkWJ7oJSFK X7KZd0