Calculate area with read and function
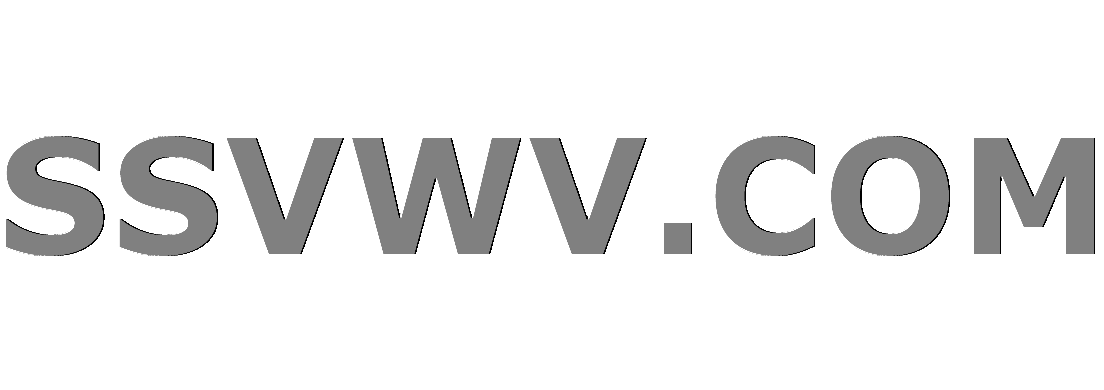
Multi tool use
up vote
1
down vote
favorite
I intend to calculate area of a circle.
#! /usr/local/bin/bash
read -p "Enter a radius: "
area () {
a=$(( 3 * $REPLY * 2 ))
return $a
}
echo $(area)
Run but return nothing
$ bash area.sh
Enter a radius: 9
Then to refactor it by quoting
#! /usr/local/bin/bash
read -p "Enter a radius: " radius
area (radius) {
a=$(( 3 * $radius * 2 ))
return "$a"
}
echo "$(area)"
It still not work properly.
bash area.sh
Enter a radius: 9
area.sh: line 3: syntax error near unexpected token `radius'
area.sh: line 3: `area (radius) {'
How to do such a calculation?
bash
add a comment |
up vote
1
down vote
favorite
I intend to calculate area of a circle.
#! /usr/local/bin/bash
read -p "Enter a radius: "
area () {
a=$(( 3 * $REPLY * 2 ))
return $a
}
echo $(area)
Run but return nothing
$ bash area.sh
Enter a radius: 9
Then to refactor it by quoting
#! /usr/local/bin/bash
read -p "Enter a radius: " radius
area (radius) {
a=$(( 3 * $radius * 2 ))
return "$a"
}
echo "$(area)"
It still not work properly.
bash area.sh
Enter a radius: 9
area.sh: line 3: syntax error near unexpected token `radius'
area.sh: line 3: `area (radius) {'
How to do such a calculation?
bash
1
The script you're running and the script you have shown as seem to be different. (There is noarea (radius) {
in your script.) Double check whatever it is you're running. Also,return
sets the exit status of the function, whereas$(area)
uses the output of the function. These are different.
– muru
Apr 10 at 2:18
ty. I modified the question.
– JawSaw
Apr 10 at 2:26
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I intend to calculate area of a circle.
#! /usr/local/bin/bash
read -p "Enter a radius: "
area () {
a=$(( 3 * $REPLY * 2 ))
return $a
}
echo $(area)
Run but return nothing
$ bash area.sh
Enter a radius: 9
Then to refactor it by quoting
#! /usr/local/bin/bash
read -p "Enter a radius: " radius
area (radius) {
a=$(( 3 * $radius * 2 ))
return "$a"
}
echo "$(area)"
It still not work properly.
bash area.sh
Enter a radius: 9
area.sh: line 3: syntax error near unexpected token `radius'
area.sh: line 3: `area (radius) {'
How to do such a calculation?
bash
I intend to calculate area of a circle.
#! /usr/local/bin/bash
read -p "Enter a radius: "
area () {
a=$(( 3 * $REPLY * 2 ))
return $a
}
echo $(area)
Run but return nothing
$ bash area.sh
Enter a radius: 9
Then to refactor it by quoting
#! /usr/local/bin/bash
read -p "Enter a radius: " radius
area (radius) {
a=$(( 3 * $radius * 2 ))
return "$a"
}
echo "$(area)"
It still not work properly.
bash area.sh
Enter a radius: 9
area.sh: line 3: syntax error near unexpected token `radius'
area.sh: line 3: `area (radius) {'
How to do such a calculation?
bash
bash
edited Apr 10 at 2:25
asked Apr 10 at 2:02


JawSaw
425312
425312
1
The script you're running and the script you have shown as seem to be different. (There is noarea (radius) {
in your script.) Double check whatever it is you're running. Also,return
sets the exit status of the function, whereas$(area)
uses the output of the function. These are different.
– muru
Apr 10 at 2:18
ty. I modified the question.
– JawSaw
Apr 10 at 2:26
add a comment |
1
The script you're running and the script you have shown as seem to be different. (There is noarea (radius) {
in your script.) Double check whatever it is you're running. Also,return
sets the exit status of the function, whereas$(area)
uses the output of the function. These are different.
– muru
Apr 10 at 2:18
ty. I modified the question.
– JawSaw
Apr 10 at 2:26
1
1
The script you're running and the script you have shown as seem to be different. (There is no
area (radius) {
in your script.) Double check whatever it is you're running. Also, return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.– muru
Apr 10 at 2:18
The script you're running and the script you have shown as seem to be different. (There is no
area (radius) {
in your script.) Double check whatever it is you're running. Also, return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.– muru
Apr 10 at 2:18
ty. I modified the question.
– JawSaw
Apr 10 at 2:26
ty. I modified the question.
– JawSaw
Apr 10 at 2:26
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
Functions in bash do not have named parameters. You cannot do:
area (foo) { ...
function area (foo) { ...
You can do:
area () {
local radius a # set a local variable that does not leak outside the function
radius=$1 # Save the first parameter to local variable
a=$(( 3 * radius * 2 ))
echo "$a"
}
And then:
echo "$(area "$REPLY")" # use $REPLY as the first argument
Since return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.
Also, while bash does not support floating-point arithmetic, it does support exponentiation:
$ bash -c 'echo $((3 * 3 ** 2))'
27
add a comment |
up vote
5
down vote
This is a quick script taking the input of the radius then feeding it to the function of area()
then echoing out the return value. This works with bc
or binary calculator installed.
#!/bin/bash
function area(){
circ=$(echo "3.14 * $1^2" | bc)
}
#Read in radius
read -p "Enter a radius: "
#Send REPLY to function
area $REPLY
#Print output
echo "Area of a circle is $circ"
Example:
terrance@terrance-ubuntu:~$ ./circ.bsh
Enter a radius: 6
Area of a circle is 113.04
Or I have expanded on the script a little to show more of reading in a variable either from the command line or from the script itself:
#!/bin/bash
function area(){
areacirc=$(printf "3.14 * $1^2n" | bc)
diamcirc=$(printf "2 * $1n" | bc)
circcirc=$(printf "2 * 3.14 * $1n" | bc)
}
#Read in radius from command line or from read
if [[ $1 == "" ]]; then
read -p "Enter a radius: "
else
printf "Radius of a cirle is $1n"
REPLY=$1
fi
#Send REPLY to area function
area $REPLY
#Print output from variables set by area function
printf "Diameter of a circle is $diamcircn"
printf "Circumference of a circle is $circcircn"
printf "Area of a circle is $areacircn"
Example:
terrance@terrance-ubuntu:~$ ./area.bsh 6
Radius of a cirle is 6
Diameter of a circle is 12
Circumference of a circle is 37.68
Area of a circle is 113.04
or
terrance@terrance-ubuntu:~$ ./area.bsh
Enter a radius: 13
Diameter of a circle is 26
Circumference of a circle is 81.64
Area of a circle is 530.66
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "89"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2faskubuntu.com%2fquestions%2f1023491%2fcalculate-area-with-read-and-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
Functions in bash do not have named parameters. You cannot do:
area (foo) { ...
function area (foo) { ...
You can do:
area () {
local radius a # set a local variable that does not leak outside the function
radius=$1 # Save the first parameter to local variable
a=$(( 3 * radius * 2 ))
echo "$a"
}
And then:
echo "$(area "$REPLY")" # use $REPLY as the first argument
Since return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.
Also, while bash does not support floating-point arithmetic, it does support exponentiation:
$ bash -c 'echo $((3 * 3 ** 2))'
27
add a comment |
up vote
3
down vote
accepted
Functions in bash do not have named parameters. You cannot do:
area (foo) { ...
function area (foo) { ...
You can do:
area () {
local radius a # set a local variable that does not leak outside the function
radius=$1 # Save the first parameter to local variable
a=$(( 3 * radius * 2 ))
echo "$a"
}
And then:
echo "$(area "$REPLY")" # use $REPLY as the first argument
Since return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.
Also, while bash does not support floating-point arithmetic, it does support exponentiation:
$ bash -c 'echo $((3 * 3 ** 2))'
27
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
Functions in bash do not have named parameters. You cannot do:
area (foo) { ...
function area (foo) { ...
You can do:
area () {
local radius a # set a local variable that does not leak outside the function
radius=$1 # Save the first parameter to local variable
a=$(( 3 * radius * 2 ))
echo "$a"
}
And then:
echo "$(area "$REPLY")" # use $REPLY as the first argument
Since return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.
Also, while bash does not support floating-point arithmetic, it does support exponentiation:
$ bash -c 'echo $((3 * 3 ** 2))'
27
Functions in bash do not have named parameters. You cannot do:
area (foo) { ...
function area (foo) { ...
You can do:
area () {
local radius a # set a local variable that does not leak outside the function
radius=$1 # Save the first parameter to local variable
a=$(( 3 * radius * 2 ))
echo "$a"
}
And then:
echo "$(area "$REPLY")" # use $REPLY as the first argument
Since return
sets the exit status of the function, whereas $(area)
uses the output of the function. These are different.
Also, while bash does not support floating-point arithmetic, it does support exponentiation:
$ bash -c 'echo $((3 * 3 ** 2))'
27
edited Apr 10 at 2:36
answered Apr 10 at 2:30


muru
135k20289492
135k20289492
add a comment |
add a comment |
up vote
5
down vote
This is a quick script taking the input of the radius then feeding it to the function of area()
then echoing out the return value. This works with bc
or binary calculator installed.
#!/bin/bash
function area(){
circ=$(echo "3.14 * $1^2" | bc)
}
#Read in radius
read -p "Enter a radius: "
#Send REPLY to function
area $REPLY
#Print output
echo "Area of a circle is $circ"
Example:
terrance@terrance-ubuntu:~$ ./circ.bsh
Enter a radius: 6
Area of a circle is 113.04
Or I have expanded on the script a little to show more of reading in a variable either from the command line or from the script itself:
#!/bin/bash
function area(){
areacirc=$(printf "3.14 * $1^2n" | bc)
diamcirc=$(printf "2 * $1n" | bc)
circcirc=$(printf "2 * 3.14 * $1n" | bc)
}
#Read in radius from command line or from read
if [[ $1 == "" ]]; then
read -p "Enter a radius: "
else
printf "Radius of a cirle is $1n"
REPLY=$1
fi
#Send REPLY to area function
area $REPLY
#Print output from variables set by area function
printf "Diameter of a circle is $diamcircn"
printf "Circumference of a circle is $circcircn"
printf "Area of a circle is $areacircn"
Example:
terrance@terrance-ubuntu:~$ ./area.bsh 6
Radius of a cirle is 6
Diameter of a circle is 12
Circumference of a circle is 37.68
Area of a circle is 113.04
or
terrance@terrance-ubuntu:~$ ./area.bsh
Enter a radius: 13
Diameter of a circle is 26
Circumference of a circle is 81.64
Area of a circle is 530.66
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
add a comment |
up vote
5
down vote
This is a quick script taking the input of the radius then feeding it to the function of area()
then echoing out the return value. This works with bc
or binary calculator installed.
#!/bin/bash
function area(){
circ=$(echo "3.14 * $1^2" | bc)
}
#Read in radius
read -p "Enter a radius: "
#Send REPLY to function
area $REPLY
#Print output
echo "Area of a circle is $circ"
Example:
terrance@terrance-ubuntu:~$ ./circ.bsh
Enter a radius: 6
Area of a circle is 113.04
Or I have expanded on the script a little to show more of reading in a variable either from the command line or from the script itself:
#!/bin/bash
function area(){
areacirc=$(printf "3.14 * $1^2n" | bc)
diamcirc=$(printf "2 * $1n" | bc)
circcirc=$(printf "2 * 3.14 * $1n" | bc)
}
#Read in radius from command line or from read
if [[ $1 == "" ]]; then
read -p "Enter a radius: "
else
printf "Radius of a cirle is $1n"
REPLY=$1
fi
#Send REPLY to area function
area $REPLY
#Print output from variables set by area function
printf "Diameter of a circle is $diamcircn"
printf "Circumference of a circle is $circcircn"
printf "Area of a circle is $areacircn"
Example:
terrance@terrance-ubuntu:~$ ./area.bsh 6
Radius of a cirle is 6
Diameter of a circle is 12
Circumference of a circle is 37.68
Area of a circle is 113.04
or
terrance@terrance-ubuntu:~$ ./area.bsh
Enter a radius: 13
Diameter of a circle is 26
Circumference of a circle is 81.64
Area of a circle is 530.66
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
add a comment |
up vote
5
down vote
up vote
5
down vote
This is a quick script taking the input of the radius then feeding it to the function of area()
then echoing out the return value. This works with bc
or binary calculator installed.
#!/bin/bash
function area(){
circ=$(echo "3.14 * $1^2" | bc)
}
#Read in radius
read -p "Enter a radius: "
#Send REPLY to function
area $REPLY
#Print output
echo "Area of a circle is $circ"
Example:
terrance@terrance-ubuntu:~$ ./circ.bsh
Enter a radius: 6
Area of a circle is 113.04
Or I have expanded on the script a little to show more of reading in a variable either from the command line or from the script itself:
#!/bin/bash
function area(){
areacirc=$(printf "3.14 * $1^2n" | bc)
diamcirc=$(printf "2 * $1n" | bc)
circcirc=$(printf "2 * 3.14 * $1n" | bc)
}
#Read in radius from command line or from read
if [[ $1 == "" ]]; then
read -p "Enter a radius: "
else
printf "Radius of a cirle is $1n"
REPLY=$1
fi
#Send REPLY to area function
area $REPLY
#Print output from variables set by area function
printf "Diameter of a circle is $diamcircn"
printf "Circumference of a circle is $circcircn"
printf "Area of a circle is $areacircn"
Example:
terrance@terrance-ubuntu:~$ ./area.bsh 6
Radius of a cirle is 6
Diameter of a circle is 12
Circumference of a circle is 37.68
Area of a circle is 113.04
or
terrance@terrance-ubuntu:~$ ./area.bsh
Enter a radius: 13
Diameter of a circle is 26
Circumference of a circle is 81.64
Area of a circle is 530.66
This is a quick script taking the input of the radius then feeding it to the function of area()
then echoing out the return value. This works with bc
or binary calculator installed.
#!/bin/bash
function area(){
circ=$(echo "3.14 * $1^2" | bc)
}
#Read in radius
read -p "Enter a radius: "
#Send REPLY to function
area $REPLY
#Print output
echo "Area of a circle is $circ"
Example:
terrance@terrance-ubuntu:~$ ./circ.bsh
Enter a radius: 6
Area of a circle is 113.04
Or I have expanded on the script a little to show more of reading in a variable either from the command line or from the script itself:
#!/bin/bash
function area(){
areacirc=$(printf "3.14 * $1^2n" | bc)
diamcirc=$(printf "2 * $1n" | bc)
circcirc=$(printf "2 * 3.14 * $1n" | bc)
}
#Read in radius from command line or from read
if [[ $1 == "" ]]; then
read -p "Enter a radius: "
else
printf "Radius of a cirle is $1n"
REPLY=$1
fi
#Send REPLY to area function
area $REPLY
#Print output from variables set by area function
printf "Diameter of a circle is $diamcircn"
printf "Circumference of a circle is $circcircn"
printf "Area of a circle is $areacircn"
Example:
terrance@terrance-ubuntu:~$ ./area.bsh 6
Radius of a cirle is 6
Diameter of a circle is 12
Circumference of a circle is 37.68
Area of a circle is 113.04
or
terrance@terrance-ubuntu:~$ ./area.bsh
Enter a radius: 13
Diameter of a circle is 26
Circumference of a circle is 81.64
Area of a circle is 530.66
edited Dec 5 at 6:49
answered Apr 10 at 2:14


Terrance
18.6k34092
18.6k34092
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
add a comment |
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
How to encapsulate the command into function area
– JawSaw
Apr 10 at 2:27
add a comment |
Thanks for contributing an answer to Ask Ubuntu!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2faskubuntu.com%2fquestions%2f1023491%2fcalculate-area-with-read-and-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
C3czZ,wYHzHEbhfKBlMuEgC3edRpTp1e LjTH7m4Ut bw9rWEJM9H,bgRlrO2d 7FoY5T83S 90doz H6iOhcUZqX2,dSP3NkTbBuz443V
1
The script you're running and the script you have shown as seem to be different. (There is no
area (radius) {
in your script.) Double check whatever it is you're running. Also,return
sets the exit status of the function, whereas$(area)
uses the output of the function. These are different.– muru
Apr 10 at 2:18
ty. I modified the question.
– JawSaw
Apr 10 at 2:26